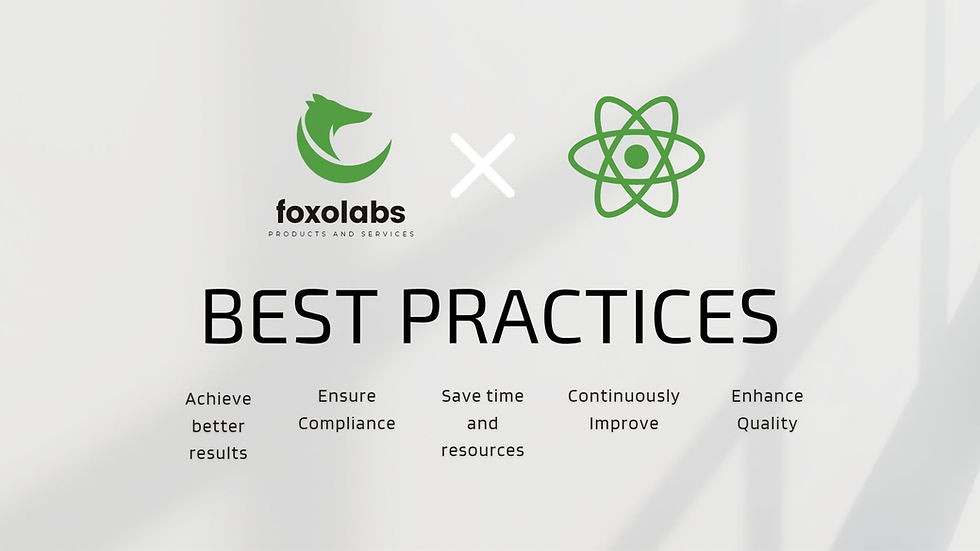
Why bother with best practices?
Best practices are a set of guidelines or recommendations that are widely accepted as the most effective and efficient way of achieving a particular goal or objective. There are several reasons why it's important to use best practices:
Achieve better results: Best practices have been tested and proven to be effective in achieving a particular goal or objective. By following best practices, you are more likely to achieve the desired results.
Save time and resources: Best practices help to streamline processes, reduce errors, and minimize waste. By following best practices, you can save time and resources that would otherwise be wasted on inefficient or ineffective methods.
Enhance quality: Best practices are designed to ensure consistency and quality in processes and outcomes. By following best practices, you can improve the quality of your work and deliver better results to your customers or clients.
Ensure compliance: Best practices often incorporate legal and regulatory requirements. By following best practices, you can ensure that you are complying with relevant laws and regulations.
Continuously improve: Best practices are constantly evolving and improving. By keeping up-to-date with best practices, you can continuously improve your processes and outcomes.
Overall, using best practices can help you to achieve better results, save time and resources, enhance quality, ensure compliance, and continuously improve.
React JS best practices
React.js is a popular JavaScript library used for building user interfaces. Here are some best practices to follow when developing with React.js:
Keep components small and focused: Break your application down into small, reusable components that each serve a specific purpose. This can help keep your code organized and make it easier to maintain.
Use a consistent folder structure and naming conventions.
Use functional components: Whenever possible, use functional components instead of class components. Functional components are easier to read, write, and test, and they perform better than class components.
Use JSX correctly: JSX is a syntax extension for JavaScript that allows you to write HTML-like syntax within your JavaScript code. Use JSX correctly by properly closing tags, using self-closing tags for empty elements, and avoiding nesting too deeply.
Use React hooks: React hooks are a new feature introduced in React 16.8 that allow you to use state and other React features in functional components. Using hooks can simplify your code and make it easier to understand and maintain.
Use a state management library: As your application grows in complexity, you may need a state management library like Redux or MobX to manage the state of your application. Using a state management library can make it easier to manage complex state and improve performance.
Optimize performance: Performance is an important consideration when building applications with React.js. Use techniques like code splitting, lazy loading, and memoization to optimize the performance of your application.
Use functional purity: Functional purity means that a function should always return the same output for the same input, without any side effects. When possible, write pure functions in your React.js application to simplify your code and make it easier to test.
Use CSS modules: CSS modules allow you to write modular CSS for your React.js components. Using CSS modules can help avoid naming collisions and make it easier to maintain your CSS code.
Use prop drilling sparingly: Prop drilling is the practice of passing props down through multiple levels of components. While it can be useful in some cases, prop drilling can make your code harder to read and maintain. Consider using a state management library like Redux or MobX to manage state instead.
Use React.Fragment: React.Fragment is a built-in feature of React.js that allows you to group a list of children without adding extra nodes to the DOM. Using React.Fragment can help keep your code clean and avoid unnecessary markup.
Use shouldComponentUpdate: shouldComponentUpdate is a lifecycle method in React.js that allows you to optimize your component rendering. Use shouldComponentUpdate to prevent unnecessary re-renders and improve the performance of your application.
Use PureComponent: PureComponent is a component class in React.js that implements shouldComponentUpdate with a shallow props and state comparison. Use PureComponent to optimize the rendering of your components and improve the performance of your application.
Use defaultProps for optional props: defaultProps is a feature of React.js that allows you to set default values for props. Use defaultProps for optional props to provide a default value when one is not provided.
Use PropTypes for type checking: PropTypes is a feature of React.js that allows you to specify the type of props that a component expects. Use PropTypes to catch errors early and improve the robustness of your application.
Use async/await for asynchronous code: async/await is a new feature in JavaScript that makes it easier to write asynchronous code. Use async/await instead of callbacks or Promises for cleaner, more readable code.
Use React.memo for performance optimization: React.memo is a higher-order component in React.js that can be used to memoize the result of a component based on its props. Use React.memo to prevent unnecessary re-renders and optimize the performance of your application.
Use React.lazy for code splitting: React.lazy is a feature of React.js that allows you to lazily load a component when it is needed. Use React.lazy to improve the performance of your application by loading only the components that are needed.
Use React.createContext for shared state: React.createContext is a feature of React.js that allows you to share state between components without having to pass props down through the component tree. Use React.createContext to simplify your code and avoid prop drilling.
Use short-circuit evaluation: Short-circuit evaluation is a technique that can be used to conditionally render components in React.js. Use short-circuit evaluation instead of ternary operators or if statements for cleaner, more concise code.
Use arrow functions for event handlers: Arrow functions are a convenient way to bind the this keyword to the current component instance in React.js event handlers. Use arrow functions instead of binding this in the constructor or using a separate method.
Use keys when rendering lists: When rendering lists in React.js, use the key prop to identify each item. Using keys can help React.js identify which items have changed and improve performance.
Use CSS-in-JS libraries: CSS-in-JS libraries like styled-components and Emotion allow you to write CSS directly in your JavaScript code. Using a CSS-in-JS library can help avoid naming collisions and make it easier to maintain your CSS code.
Use the spread operator for props: The spread operator can be used to pass props to a component in a cleaner, more concise way. Use the spread operator to avoid manually passing props one-by-one and improve the readability of your code.
Use the ES6 import/export syntax: The ES6 import/export syntax can be used to import and export modules in your React.js application. Use the ES6 syntax instead of CommonJS require and module.exports for cleaner, more modern code.
Use ESLint and Prettier for code formatting: ESLint and Prettier are two popular tools for enforcing code formatting in your React.js application. Use ESLint and Prettier to ensure consistent code formatting and improve the readability of your code.
Use propTypes.shape for complex props: propTypes.shape is a feature of React.js that allows you to define the shape of a complex prop object. Use propTypes.shape to improve the robustness of your code and catch errors early.
Use propType.oneOfType for mixed types: propTypes.oneOfType is a feature of React.js that allows you to define a prop as one of several possible types. Use propTypes.oneOfType to handle cases where a prop can be one of several different types.
Use the useMemo hook for expensive computations: The useMemo hook is a feature of React.js that allows you to memoize the result of a function based on its inputs. Use useMemo to prevent expensive computations from being re-run unnecessarily and improve the performance of your application.
Use stateless functional components where possible: Stateless functional components are simpler and easier to reason about than class components. Use stateless functional components where possible for cleaner, more concise code.
Use React DevTools for debugging: React DevTools is a browser extension that allows you to inspect and debug your React components. Use React DevTools to identify and resolve issues in your application.
Use React Router for client-side routing: React Router is a popular library for client-side routing in React.js applications. Use React Router to manage navigation and state between different pages in your application.
Use the useEffect hook for side effects: The useEffect hook is a feature of React.js that allows you to perform side effects such as fetching data or updating the DOM. Use useEffect to keep your components clean and focused on their main responsibility.
Use the useCallback hook to optimize performance: The useCallback hook can be used to memoize a function and prevent unnecessary re-renders in your React components.
Use TypeScript for static typing: TypeScript is a typed superset of JavaScript that can help you catch errors early and improve the maintainability of your code. Use TypeScript to add static typing to your React.js application.
By following these best practices, you can write clean, maintainable code that is easy to understand and optimize for performance.
Security best practices for React.js
Use HTTPS: Use HTTPS instead of HTTP to ensure that data transmitted between the client and server is encrypted and secure.
Avoid storing sensitive information in the client-side code: Sensitive information such as passwords and API keys should not be stored in the client-side code as it can be easily accessed by attackers.
Implement proper authentication and authorization: Implement proper authentication and authorization mechanisms to ensure that only authorized users can access sensitive data and functionality.
Use input validation: Use input validation to ensure that user input is in the correct format and prevent attacks such as SQL injection and cross-site scripting (XSS).
Use parameterized queries: Use parameterized queries to prevent SQL injection attacks by ensuring that user input is treated as data rather than code.
Use third-party libraries cautiously: Use third-party libraries cautiously and ensure that they are up-to-date and secure to prevent vulnerabilities in your application.
Avoid using eval() and Function() for dynamic code execution: Avoid using eval() and Function() for dynamic code execution as it can be a security risk by allowing attackers to inject malicious code.
Use Content Security Policy (CSP): Use Content Security Policy (CSP) to restrict the sources from which scripts, stylesheets, and other resources can be loaded.
Implement rate limiting: Implement rate limiting to prevent attackers from brute-forcing authentication or flooding your server with requests.
Keep your dependencies up-to-date: Keep your dependencies up-to-date to ensure that your application is not vulnerable to known security issues in third-party libraries.
By following these and other security best practices, you can help ensure that your React.js application is secure and protected against common attacks.
Here are some additional best practices to consider when building a React.js application with a backend:
Use a scalable backend architecture: Choose a backend architecture that can scale as your application grows. Common options include serverless architecture, microservices architecture, and monolithic architecture.
Implement a RESTful API: Use a RESTful API to communicate between your frontend and backend. A RESTful API follows a set of architectural constraints and principles to ensure interoperability between different systems.
Implement error handling: Implement error handling in your backend to provide informative error messages to the frontend and improve the user experience.
Use environment variables for configuration: Use environment variables to store configuration values such as API keys and database credentials. This can help you avoid hardcoding sensitive information in your code.
Use a database that is well-suited to your needs: Choose a database that is well-suited to your needs and can handle the data requirements of your application. Popular options include MySQL, PostgreSQL, MongoDB, and Firebase.
Implement caching and pagination for better performance: Implement caching and pagination to improve the performance of your backend and reduce the load on your server.
Use a load balancer for high availability: Use a load balancer to distribute traffic between multiple servers and improve the availability of your application.
Use a content delivery network (CDN) for faster content delivery: Use a CDN to cache and deliver static content such as images and videos from servers located closer to the user.
Implement logging and monitoring: Implement logging and monitoring in your backend to track errors, monitor performance, and identify opportunities for optimization.
Implement security measures: Implement security measures such as encryption, access control, and rate limiting to protect your backend against attacks and ensure the confidentiality and integrity of your data.
By following these and other best practices, you can build a robust and scalable React.js application with a backend that is secure, performant, and easy to maintain.
Here are some best practices to consider when testing your React.js application:
Use a testing framework: Use a testing framework such as Jest, Mocha, or Enzyme to automate your tests and simplify the testing process.
Write unit tests for individual components: Write unit tests for each individual component in your application to ensure that they function correctly and as expected.
Write integration tests for the entire application: Write integration tests for the entire application to ensure that all components work together as expected and that the application as a whole functions correctly.
Use mock data and dependencies: Use mock data and dependencies to simulate different scenarios and test edge cases in your application.
Use snapshot testing for UI components: Use snapshot testing to compare the current state of a UI component with a saved snapshot to ensure that there are no unintended changes.
Use code coverage tools: Use code coverage tools such as Istanbul to measure the percentage of code that is covered by your tests.
Test performance and scalability: Test the performance and scalability of your application to ensure that it can handle a large number of users and that it responds quickly to user interactions.
Automate testing with continuous integration (CI): Use a continuous integration (CI) tool such as Travis CI or CircleCI to automate your testing process and ensure that tests are run every time code is pushed to the repository.
Test for accessibility: Test your application for accessibility to ensure that it can be used by people with disabilities.
Test for security: Test your application for security vulnerabilities to ensure that it is secure and that user data is protected.
By following these and other testing best practices, you can ensure that your React.js application is reliable, secure, and easy to maintain.
Here are some best practices to consider when deploying your React.js application:
Choose a reliable hosting service: Choose a hosting service that is reliable and can handle the traffic and data requirements of your application. Some popular options include AWS, Google Cloud, Heroku, and Netlify.
Use a CDN for faster content delivery: Use a content delivery network (CDN) to cache and deliver static content such as images and videos from servers located closer to the user.
Use HTTPS to secure communication: Use HTTPS to secure communication between the client and server and protect user data from interception and tampering.
Use version control and continuous integration: Use version control such as Git and a continuous integration (CI) tool such as Jenkins or Travis CI to automate the deployment process and ensure that the latest code is deployed.
Use environment variables for configuration: Use environment variables to store configuration values such as API keys and database credentials. This can help you avoid hardcoding sensitive information in your code.
Use a reverse proxy for load balancing: Use a reverse proxy such as Nginx or Apache to distribute traffic between multiple servers and improve the availability and performance of your application.
Implement logging and monitoring: Implement logging and monitoring in your application to track errors, monitor performance, and identify opportunities for optimization.
Use a containerization platform: Use a containerization platform such as Docker to simplify deployment and ensure consistency across different environments.
Use a load testing tool: Use a load testing tool such as Apache JMeter or Locust to simulate traffic and test the performance and scalability of your application.
Implement backups and disaster recovery: Implement backups and disaster recovery measures to ensure that your application and data are protected against data loss and downtime.
By following these and other deployment best practices, you can ensure that your React.js application is reliable, secure, and performs well in production.
Here are some best practices to consider when styling your React.js application:
Use a CSS preprocessor: Use a CSS preprocessor such as Sass or Less to improve code organization and maintainability.
Use a CSS-in-JS library: Use a CSS-in-JS library such as styled-components, emotion, or JSS to manage CSS styles within the JavaScript code.
Use a consistent naming convention: Use a consistent naming convention for CSS classes and IDs to make your code more organized and readable.
Use a modular approach: Use a modular approach to style your components, where each component has its own stylesheet. This can help you avoid CSS conflicts and make your code more maintainable.
Use a design system: Use a design system to create a consistent look and feel across your application and ensure that styles are applied consistently.
Use responsive design: Use responsive design to ensure that your application looks good and functions well on different screen sizes and devices.
Use accessibility guidelines: Use accessibility guidelines such as WCAG to ensure that your application is accessible to users with disabilities.
Use color palettes: Use color palettes to create a consistent color scheme across your application and ensure that colors are used consistently.
Use typography guidelines: Use typography guidelines to create a consistent typography style across your application and ensure that typography is legible and easy to read.
Use a style guide: Use a style guide to document your styling guidelines and ensure that they are followed consistently across your application.
By following these and other styling best practices, you can ensure that your React.js application is visually appealing, organized, and easy to maintain.
Logging and monitoring
Logging and Monitoring are essential components of any production-grade application, including React.js frontend applications. Here are some best practices to consider when implementing logging and monitoring in your React.js application:
Use a logging library: Use a logging library such as Winston or Bunyan to log errors and events in your application.
Implement error boundaries: Implement error boundaries in your components to catch and handle errors that occur during rendering.
Use performance monitoring tools: Use performance monitoring tools such as React Profiler or the Chrome DevTools Performance tab to identify performance bottlenecks and improve application speed.
Use a centralized logging solution: Use a centralized logging solution such as Elasticsearch or Splunk to collect and analyze logs from different parts of your application.
Implement distributed tracing: Implement distributed tracing using tools such as OpenTelemetry or Zipkin to track requests across different services and identify performance issues.
Monitor user behavior: Monitor user behavior using tools such as Google Analytics or Mixpanel to understand how users interact with your application and identify opportunities for improvement.
Implement alerting: Implement alerting using tools such as PagerDuty or Datadog to notify you when critical errors or performance issues occur.
Monitor dependencies: Monitor dependencies using tools such as Node.js Monitoring or New Relic to identify issues with external dependencies and third-party APIs.
Implement A/B testing: Implement A/B testing using tools such as Optimizely or VWO to test different versions of your application and identify the best performing option.
Use continuous integration and deployment: Use continuous integration and deployment (CI/CD) tools such as CircleCI or Jenkins to automate the logging and monitoring process and ensure that the latest code is deployed to production.
By implementing these and other logging and monitoring best practices, you can ensure that your React.js application is reliable, performant, and easy to maintain in production.
Comments