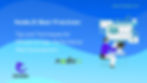
Why bother with best practices?
Best practices are a set of guidelines or recommendations that are widely accepted as the most effective and efficient way of achieving a particular goal or objective. There are several reasons why it's important to use best practices:
Achieve better results: Best practices have been tested and proven to be effective in achieving a particular goal or objective. By following best practices, you are more likely to achieve the desired results.
Save time and resources: Best practices help to streamline processes, reduce errors, and minimize waste. By following best practices, you can save time and resources that would otherwise be wasted on inefficient or ineffective methods.
Enhance quality: Best practices are designed to ensure consistency and quality in processes and outcomes. By following best practices, you can improve the quality of your work and deliver better results to your customers or clients.
Ensure compliance: Best practices often incorporate legal and regulatory requirements. By following best practices, you can ensure that you are complying with relevant laws and regulations.
Continuously improve: Best practices are constantly evolving and improving. By keeping up-to-date with best practices, you can continuously improve your processes and outcomes.
Overall, using best practices can help you to achieve better results, save time and resources, enhance quality, ensure compliance, and continuously improve.
Node JS best practices
General
Use the latest version of Node.js. Download here
Package, Environment Configurations and Version Control
Use package-lock.json for consistent package versions
Use Yarn or npm for package management
Use .env files for environment variables
Use process.env for environment variables in production
Use environment-specific configuration files
Use JSON for configuration files
Use a .gitignore file to exclude unnecessary files from version control
Use Nodemon for development server
Code Style and Coding patterns
Use a linter for consistent code style
Use ESLint with the Airbnb style guide
Use semicolons to terminate statements
Use double quotes for strings
Use === and !== instead of == and !=
Use const for variable declaration
Use let for mutable variables
Use destructuring for object and array assignment
Use arrow functions for concise function syntax
Use async/await for asynchronous code
Use Promises for asynchronous code
Use callbacks for asynchronous code when necessary
Use event emitters for custom events
Use the EventEmitter class for custom events
Utilities
Use Bluebird for Promise utilities
Use Lodash for utility functions
Use Moment for date and time manipulation
Use Joi for input validation
Debugging & Logging in Code
Use the util module for debugging
Use the console module for logging
Use Winston for logging in production
Use Bunyan for structured logging
Use Morgan for HTTP request logging
Logging, Monitoring, Error Tracking and Log Analysis
Use New Relic for application monitoring
Use Datadog for application monitoring
Use Sentry for error tracking
Use Rollbar for error tracking
Use Loggly for centralized logging
Use Papertrail for centralized logging
Use Prometheus for monitoring
Use Grafana for monitoring visualization
Use Splunk for log analysis
Use ELK Stack for log analysis
Use Kibana for log visualization
Use Nagios for system monitoring
Security & Frameworks
Use Helmet for securing HTTP headers
Use CORS for cross-origin resource sharing
Use Express for web applications
Use Hapi for web applications
Use Koa for web applications
Use Fastify for web applications
Real-time Communication
Use Socket.io for real-time communication
Use WebSocket for real-time communication
Scaling & Process Management
Use the cluster module for scaling
Use pm2 for process management
Testing and Code Coverage
Use Chai for assertion library
Use Sinon for mocking and stubbing
Use Supertest for HTTP testing
Use Mocha for testing framework
Use Jest for testing framework
Use Istanbul for code coverage
Documentation
Tool: Use Swagger for API documentation
Use JSDoc comments: JSDoc is a popular documentation tool for JavaScript, and it can be used to generate documentation for Node.js projects. JSDoc comments can be added to functions, variables, and classes to provide information about their purpose, inputs, and outputs.
Document public APIs: When documenting a Node.js project, it's important to focus on the public APIs that will be used by other developers. These APIs should be well-documented, including information on their purpose, inputs, and outputs.
Use a consistent format: Use a consistent format for documenting your code. This can help ensure that your documentation is easy to read and understand.
Keep documentation up-to-date: Documentation should be updated whenever changes are made to the code. Keeping documentation up-to-date can help prevent confusion and errors when using the code.
Include examples: Including examples in your documentation can help developers understand how to use your code. Examples should cover common use cases and provide clear instructions on how to use the code.
Organize documentation: Organize your documentation in a logical and consistent manner. This can help make it easier for developers to find the information they need.
Consider using a documentation generator: There are several documentation generators available for Node.js, including JSDoc and DocPad. Using a documentation generator can help automate the process of creating and maintaining documentation.
Database Migration
Use Sequelize for SQL database ORM
Use Mongoose for MongoDB ORM
Caching, Proxy and Load Balancing
Use Redis for caching
Use Nginx for reverse proxy and load balancing
Authentication and Encryption
Use Passport for authentication
Use OAuth for third-party authentication
Use JWT for stateless authentication
Use bcrypt for password hashing
Use crypto for encryption
Cloud Hosting
Use AWS for cloud hosting
Use Google Cloud for cloud hosting
Use Heroku for cloud hosting
Use Azure for cloud hosting
Containerization & CI/CD
Use Docker for containerization
Use Kubernetes for container orchestration
Use Terraform for infrastructure as code
Use Ansible for configuration management
Use CircleCI for continuous integration
Use Jenkins for continuous integration
Use Travis CI for continuous integration
Use GitHub Actions for continuous integration